Here is a small screen shot
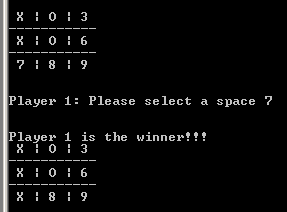
The Logic
I knew I needed some sort of text to display the tictactoe board. However I didnt know how to differentiate between each square. I finally decided to use numbers. The start up board will look like this:
1 | 2 | 3
----------
4 | 5 | 6
----------
7 | 8 | 9
This allowed me to create the player input, which would be the space number. Then I would replace the space number with the check mark, “X” or “O”.
The other problem I initally had was how was I going to check for a winner. After many drawings and psuedo code, I decided to keep the winning combinations in an array, grouped in three and then iterate through.
The winning combinations are: 123, 456, 789, 147, 258, 369, 159, 357.
Next I wondered how I would keep track of how many spaces were left? Well that was easy, just create a spaces array. After this I knew I had my groundwork set and began coding.
The code
Here we will load the initial variable values that we need.
# Load the board board = " 1 | 2 | 3n-----------n 4 | 5 | 6n-----------n 7 | 8 | 9" # Set the winning combinations. Paired off in threes # For example: 123 is a winning combo. So is 147. checkboard=[1,2,3,4,5,6,7,8,9,1,4,7,2,5,8,3,6,9,1,5,9,3,5,7] # Set Space Holder spaces=range(1,10)
Next let’s start the game and follow the flow of the code from there. We want to use a while loop because we want the game to go back and forth between the players, until someone wins or no more spaces left.
#Start Game while True: player = len(spaces)%2 +1 if player == 1: player = 2 else: player =1 print "\n\n" + board
Lets break it down. First we check who is going first by using len(spaces)%2 +1. This will return either 1 or 2 depending on whether the length of array ’spaces’ is odd or even. However, the initial length of the array is odd since there are nine space, meaning that player 2 would go first, but we want player 1 to go first. We fix this with the if statement that follows.
Now we want to request from the player what space they want to pick. The playerinput() function will handle this for us.
def playerinput(player): try: key = int(raw_input('\n\nPlayer ' + str(player) + ': Please select a space ')) except ValueError: print "Invalid Space" key = playerinput(player) return key
Notice that we pass the ‘player’ variable so that when we ask for input, we know which player to ask. We then ask the player to input a number representing the space. However, what if the player does not input a number? What happens to the program? That is why we have the ‘try’ and ‘except’. We try to ask the player for a number, however if a number is not inputted, then a ‘ValueError’ occurs and we then hit the except case to handle it and ask for another. When a number is finally inputted we return that number as ‘key’.
Once we have the space number, we need to make sure it is not in use and if not, then we need to set it as used. In the main while loop we call to to movehandler function:
board,status =moveHandler(board,spaces,checkboard,player,key)
The moveHanlder function looks like this:
def moveHandler(board,spaces,checkboard,player,n): # set X for player one, or O for player 2 if player==1: check="X" else: check="O" # Check if block is used. while spaces.count(n)==0: print "\nInvalid Space" n=playerinput(player) # Remove block from spaces array spaces=spaces.remove(n) # Replace block with check mark in board board=board.replace(str(n),check) # Replace space with check mark in checkboard array for c in range(len(checkboard)): if checkboard[c]==n: checkboard[c]=check # Run the checkwinner function status = checkwinner(checkboard,check) return board,status
The first thing this function does is check which player is up in order to assign x’s and o’s to the ‘check’ variable. After that, we need to check to see if the inputted space was already in use. So we check the spaces array for that number using the spaces.count(n) loop. If it is in use, we ask the player to input a number again. If the number is not in use then we remove it with the spaces array with spaces.remove(n). Also, we need to place a check mark, either x or o onto that spot on the board. We do this with the string replace command, board.replace(str(n),check).
Next we mark off the x or o in the checkboard array whereever that space is. Finally we need to check for a winner, so we call the checkwinner function here:
def checkwinner(checkboard,check): # Set the array element variables a,b,c=0,1,2 #Loop through checkboard to check winner while a<=21: combo = [checkboard[a],checkboard[b],checkboard[c]] # If we have three 'X' or 'O' we have a winner if combo.count(check) == 3: status =1 break else: status =0 # Iterate to the next combo a+=3 b+=3 c+=3 # Return status of the game return status
This function cycles through the checkboard array and looks for three x’s or o’s in a group set of 3 which will mean there is a winner.
The code ends with then end of the main while loop. We check if there is a winner, if not we check to see if there are spaces left, if there are then we continue.
if status == 1: print '\n\nPlayer ' + str(player) + ' is the winner!!!' print board break elif len(spaces)==0: print "No more spaces left. Game ends in a TIE!!!" print board break else: continue
Well thats it for today’s tutorial on Python. For more information about python please visit http://www.python.org
The full script can be seen here:
# PyTicTacToe# © 2007 by Patrick O'Brien# [url="http://www.obtown.com"]http://www.obtown.com[/url]# Load the boardboard = " 1 | 2 | 3\n-----------\n 4 | 5 | 6\n-----------\n 7 | 8 | 9"# Set the winning combinations. Paired off in threes# For example: 123 is a winning combo. So is 147. checkboard=[1,2,3,4,5,6,7,8,9,1,4,7,2,5,8,3,6,9,1,5,9,3,5,7]# Set Space Holderspaces=range(1,10)# movehandler function# This function takes the players choice and makes sure it # has not been used. If not, set the move. def moveHandler(board,spaces,checkboard,player,n): # set X for player one, or O for player 2 if player==1: check="X" else: check="O" # Check if block is used. while spaces.count(n)==0: print "\nInvalid Space" n=playerinput(player) # Remove block from spaces array spaces=spaces.remove(n) # Replace block with check mark in board board=board.replace(str(n),check) # Replace space with check mark in checkboard array for c in range(len(checkboard)): if checkboard[c]==n: checkboard[c]=check # Run the checkwinner function status = checkwinner(checkboard,check) return board,status# Checkwinner function# This function checks whether or not a player has a winning# combination based on the checkboard array.def checkwinner(checkboard,check): # Set the array element variables a,b,c=0,1,2 #Loop through checkboard to check winner while a<=21: combo = [checkboard[a],checkboard[b],checkboard[c]] # If we have three 'X' or 'O' we have a winner if combo.count(check) == 3: status =1 break else: status =0 # Iterate to the next combo a+=3 b+=3 c+=3 # Return status of the game return status# def playerinput(player): try: key = int(raw_input('\n\nPlayer ' + str(player) + ': Please select a space ')) except ValueError: print "Invalid Space" key = playerinput(player) return key #Start Gamewhile True: player = len(spaces)%2 +1 if player == 1: player = 2 else: player =1 print "\n\n" + board key = playerinput(player) board,status =moveHandler(board,spaces,checkboard,player,key) if status == 1: print '\n\nPlayer ' + str(player) + ' is the winner!!!' print board break elif len(spaces)==0: print "No more spaces left. Game ends in a TIE!!!" print board break else: continue