#include <signal.h> #include <stdio.h> #include <string.h> #include <sys/types.h> #include <unistd.h> sig_atomic_t count = 0; void handler (int signal_number) { count++; } void handler2 (int signal_number) { if(count >= 10) { printf("You have counted too many digits.\n" "You wanted %d and you can only use 9.\n" "I can't just grow an extra finger or toe people. Start over.\n",count); count = 0; } else { printf("%d\n",count); count = 0; } } void handler3 (int signal_number) { printf("Liar! It is not dead (just wounded).\n") ; } int main(int argc, char ** argv) { struct sigaction sa1, sa2, sa3; int pid; memset (&sa1, 0, sizeof (sa1)); sa1.sa_handler = &handler; sigaction(SIGUSR1,&sa1,NULL); memset (&sa2,0,sizeof(sa2)); sa2.sa_handler = &handler2; sigaction(SIGUSR2,&sa2,NULL); memset (&sa3,0,sizeof(sa2)); sa3.sa_handler = &handler3; sigaction(SIGCHLD,&sa3,NULL); pid = fork(); if (pid != 0) { for(;;) { sleep(1000); //printf("I'm awake, where's the coffee\n"); } } else { for(;;); } wait(pid); }
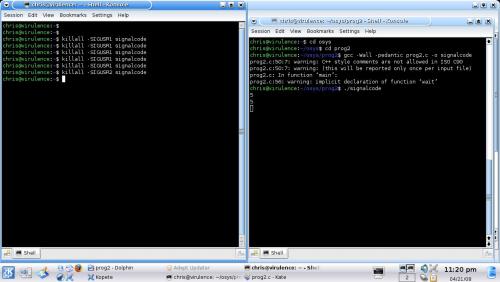
Thanks in advance!
Chris