#include <iostream> using namespace std; int divisibleBy (int x) { if (x == 1) { cout << x << "is divisible by five!" << endl; } else { divisibleBy (x-5/5); if (x!=1){ cout<<"x is not diviible by 5"<< endl; } if (x==1){ cout <<"x is divisible by 10"<< endl; } else if (x!=1){ cout<<"x is not divisible by 10" << endl; } return 0; } } int main() { int x; cout << "Please enter a number: " << endl; cin >> x; divisibleBy(x); return 0; }
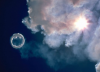
Divisible or not, nested if statements
Started by
willmon2000
, Feb 24 2012 03:11 PM
#1
Posted 24 February 2012 - 03:11 PM

#2
Posted 24 February 2012 - 03:47 PM

Hey there willmon2000,
I don't seem to understand your logic behind your "divisibleBy" function where you are comparing your "x" variable to "1" and your "x-5/5". I think what you are trying to do there is look for a remainder of a certain number when divided by another number. The best way to achieve this is by using the modulus operator to return back a remainder rather than just dividing the number. I have also neatened up your function and made it a void function since you don't seem to be returning anything of importance, here is the revised code:

Peace Out
I don't seem to understand your logic behind your "divisibleBy" function where you are comparing your "x" variable to "1" and your "x-5/5". I think what you are trying to do there is look for a remainder of a certain number when divided by another number. The best way to achieve this is by using the modulus operator to return back a remainder rather than just dividing the number. I have also neatened up your function and made it a void function since you don't seem to be returning anything of importance, here is the revised code:
#include <iostream> using namespace std; void divisibleBy(int x); int main() { int x; cout << "Please enter a number:" << endl; cin >> x; divisibleBy(x); return 0; } void divisibleBy(int x) { if (x % 5 > 0) cout << x << " is not divisible by five!" << endl; else cout << x << " is divisible by five!" << endl; if (x % 10 > 0) cout << x << " is not divisible by ten!" << endl; else cout << x << " is divisible by ten!" << endl; }Please let me know if this was what you were looking for and if there is anything else you need

Peace Out

#3
Posted 24 February 2012 - 04:23 PM

.
Edited by willmon2000, 24 February 2012 - 04:25 PM.
Similar Topics
1 user(s) are reading this topic
0 members, 1 guests, 0 anonymous users
As Featured On:
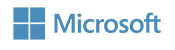
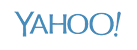
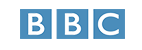
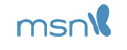


